Today I’ll show you how to run your Compose UI tests in Firebase Test Lab.
Let’s start by adding the necessary dependencies in your app/build.gradle
file.
testImplementation "junit:junit:4.13.2"
androidTestImplementation "androidx.test:runner:1.5.2"
androidTestImplementation "androidx.test:rules:1.5.0"
androidTestImplementation "androidx.compose.ui:ui-test-junit4:1.5.4"
debugImplementation "androidx.compose.ui:ui-test-manifest:1.5.4"
Also set the testInstrumentationRunner
inside the android
block.
android {
defaultConfig {
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
}
}
Now inside you androidTest
folder create a class for the UI test.
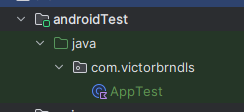
Let’s write a simple test that fails for us to verify we can run it.
class AppTest {
@get:Rule
val composeTestRule = createAndroidComposeRule<MainActivity>()
@Test
fun test() {
composeTestRule.waitUntil(10_000) { false }
}
}
Make sure your testing device is setup for UI testing. Follow these instructions if you haven’t already.
Now we can run the UI test.

As expected, it failed after about 10s. Now let’s add a simple check, this will vary from project to project.
My app has a home screen that looks like this:

So I modified my test to check for the “Intellectra” title and then press the button
@Test
fun test() {
composeTestRule.onNode(hasText("Intellectra"))
.assertIsDisplayed()
composeTestRule.onNode(hasText("Click me"))
.performClick()
}
Let’s run the test again

We can see that it passed. Now let’s use Firebase Test Lab to automate running the tests.
We’ll use Fastlane to do that. If you don’t have Fastlane installed, you can check their documentation here.
After you install Fastlane, go the your Fastlane
file and add the following code:
platform :android do
desc "Your App Name"
lane :ui_test do
build_android_app(
tasks: [
"assembleDebug",
"assembleDebugAndroidTest"
],
print_command: false
)
run_tests_firebase_testlab(
project_id: "firebase-project-id",
devices: [
{
model: "redfin", # pixel 5
version: "30"
}
],
gcloud_service_key_file: "google-services.json",
extra_options: "--use-orchestrator --environment-variables clearPackageData=true",
download_results_from_firebase: false
)
end
end
Now you have a lane that runs your UI tests on Firebase for you. To execute this lane run bundle exec fastlane ui_test
.
If you have any comments or suggestions, please reach out to me on Twitter.
Photo by Roman Mager on Unsplash